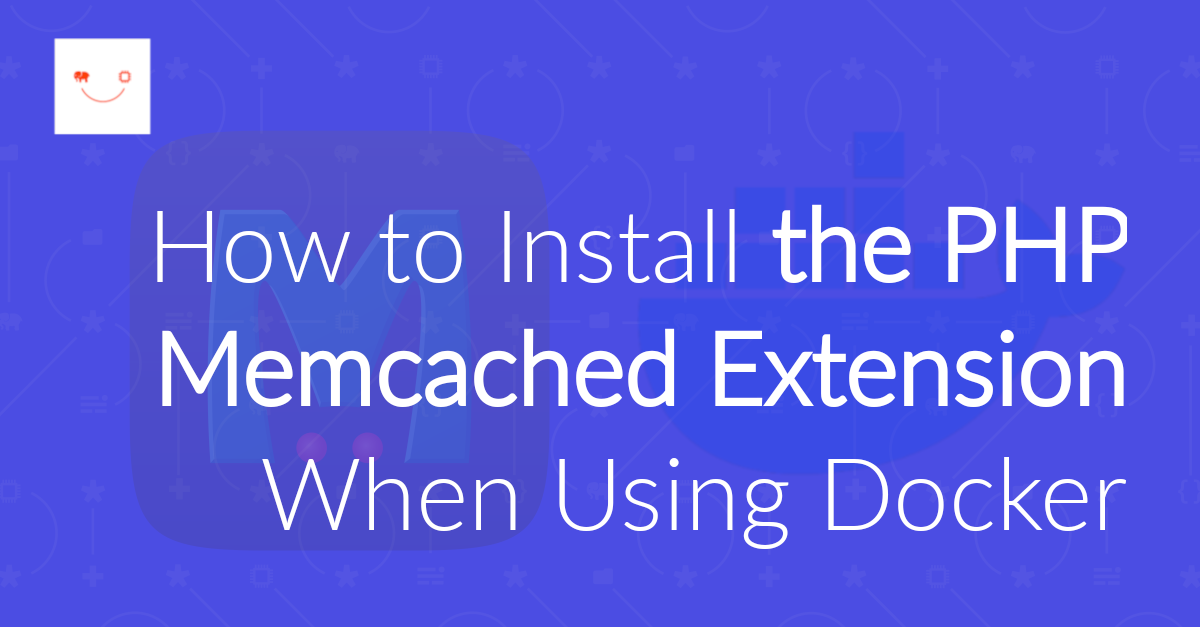
How to Install the PHP Memcached Extension When Using Docker
If you’re having trouble getting PHP’s Memcached extension working in a Docker container, this tutorial will show you how.
If you’re getting started learning PHP or want to get started, this post is for you. In it, I step you through the essential things you need and things you need to know to start your learning the right way.
When starting anything new, whether that’s a human or software development language, it’s often challenging to know where to start, what tools and other things you’ll need, how to measure your progress, and what exactly you need to learn. PHP is no exception. So, after putting quite some thought into this, here’s what I believe you need to do to learn PHP in 2025.
While there are so many things that you could start with, let’s keep this as simple and focused as possible, concentrating on the absolute essentials of:
With these in place, you’re ready to start learning PHP. However, I see them as just the initial things you’ll need.
You’ll likely want to go further with the following:
If you’re familiar with containerisation (which we all likely are) you might prefer to use either Docker (and Docker Compose) or Podman to simplify creating development environments for your PHP projects, instead of installing everything directly on your local development machine. Doing so could greatly simplify switching between versions of PHP and installing various PHP extensions.
If you are more familiar with (or prefer) Docker, make sure you check out Deploy With Docker Compose, a free book that I wrote, taking you from zero to deployed PHP app with Docker Compose. And, if you develop with Laravel, check out Laravel Sail, a light-weight command-line interface for interacting with Laravel’s default Docker development environment.
In the early days, PHP was synonymous with MySQL; according to the JetBrains 2024 State of Developer Ecosystem Report it’s the most popular choice. Despite being around in those days, I don’t remember why the two were synonymous with one another.
Actually, even at the time I don’t think I knew why, though this Quora thread may have the answer. That said, there are so many options to choose from these days, including my favourite is SQLite, as well as PostgreSQL, Microsoft SQL Server, Redis, Amazon Athena, and Couchbase.
If you really want to learn PHP properly, however, you’re going to need a number of additional things. Let’s look at what those are.
You need to know how to organise the source and related files that your project will require, such as your tests. Now, proper file organisation isn’t specific to PHP, as any good software language or project requires a high degree of proper organisation.
Gladly, PHP has PSR-4 (Autoloader) to help you manage this, which describes where to place files. I strongly encourage you to familiarise yourself with the PSR and Composer’s autoload documentation.
There are many CI/CD (Continuous Integration/Continuous Deployment) tools available that support PHP, such as GitHub Actions, Jenkins, GitLab, CircleCI, [TeamCity(https://www.jetbrains.com/teamcity/)], and Travis CI.
If you’re not familiar with the term, CI/CD tools automate building your (PHP) applications, such as running tests and all your other QA tools, and deploying them to production. Whereas you, and the rest of your team, might have run tests and static analysis, as well as deployed apps manually, doing so can be quite involved.
What’s more, it leaves open the possibility of one or more steps being missed, leading to failed deployments (and unhappy users) as well as requires unnecessary effort, reducing the time that the development team can be maintaining the code.
So, familiarise yourself with a CI/CD tool, making sure your know the basics of how to configure it, as well as what its key commands are.
Let’s take my preferred tool, GitHub Actions, as an example. To configure it, you use Yaml files and store them in a directory named .github/workflows in your project. By doing so, no one needs to learn a new UI for configuring project integration, and the configuration is kept alongside the code that it builds.
Here’s a simple example of a Yaml configuration file:
name: PHP QA Suite
on:
pull_request:
branches: [ "main" ]
permissions:
contents: read
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Validate composer.json and composer.lock
run: composer validate --strict
- name: Cache Composer packages
id: composer-cache
uses: actions/cache@v3
with:
path: vendor
key: ${{ runner.os }}-php-${{ hashFiles('**/composer.lock') }}
restore-keys: |
${{ runner.os }}-php-
- name: Install dependencies
run: composer install --prefer-dist --no-progress
- name: Run QA suite
run: composer run-script cs-check
- name: Run test suite
run: composer run-script test
Stepping through it from top to bottom, the workflow is named “PHP QA Suite”, as it runs the QA tools on the project’s code, but doesn’t deploy the code. Then, it provides instructions for when to run the workflow. In this case, they’ll be run when pull requests are made against the main branch.
Then comes the jobs that will be run in the workflow. In the above example there is only one, named “build”, which has six steps:
This should be a given, but perhaps I’m biased, but I believe that you need to know what Composer is, why you should use it, and how to use it.
If you’ve not used Composer before, then start with the Basic Usage section of the documentation. This section shows the essentials of composer.json and how to install and update project dependencies, as well as autoloading.
Then, if you’d like to dive into particular areas, I’ve written a series of hands-on tutorials for your reading pleasure.
PHP’s up to version 8.4, so it has a lot of functionality and features. While you could take the time to learn about all of it, and I strongly recommend you diving deep into it, start with the 8.x release. To me, it’s one of the most exciting releases because of features such as:
Your best source of information is the PHP documentation; you’ll always find a link to the latest changes on the home page. However, two other great sources are PHP.Watch and stitcher.io. And, if you want to know what’s coming in future releases, check out the PHP RFCs (Requests for Comment).
I know that I don’t speak for everyone when I say that automated testing is an integral part of software development – not something that you add on or bolt on later.
I’ve worked in development shops where testing was the developer checking their work in their browser or with curl requests after they’d written it, and if it worked, it was committed and pushed to production.
I’m sure that that works for some people. But I’m not one of them. For me, tests need to be automated so that they’re repeatable, provide additional knowledge about the application – and so much more. But, I’ll leave that debate for another date and another post.
For now, you need to know the following:
There are many different types of testing used in modern PHP development, including unit, functional, integration, and smoke testing. Then, at a higher level, there are different testing methodologies, such as Test-Driven Development (TDD), and Behavior-Driven Development (BDD).
If you’re coming to testing for the first time, or are quite new to it, you could easily feel quite confused as to where to start. So, my advice is to start with unit tests, then progress from there. To do that, start with PHPUnit, one of the oldest – if not the oldest – testing framework for PHP.
Once you’re familiar with PHPUnit, then, if you need to, branch out from there, to other frameworks and tools. You’re not short of options. such as there’s Pest, Behat, Codeception, and Selenium to name but a few.
While PHP can be written in both a functional and an OOP-style, the latter is far more prevalent in my experience. There’s a bit to learn if you’re new to the topic, so here are some resources to get you started:
I would feel deeply remiss if I didn’t provide additional resources for learning PHP, so that, as the saying goes, you can help those who help themselves. So, here are a host of resources that I’m using in my PHP journey. I hope that they help you as well.
I’m a strong believer that we learn better with and from others. So, here’s a set of great groups and forums filled with knowledgeable, experienced PHP developers who are ready to help one another:
If you prefer to learn by listening, or learn on the go such as when driving, riding, walking, or commuting, here is a short selection of podcasts to add to your collection:
If you’re looking for some great PHP-focused/experienced developers to follow, here is a small selection of the ones that I am familiar with.
If you’re someone who prefers a good read and to learn by reading, then here is a small selection of books to get you going.
Do you prefer to learn by watching great content? Then here are some solid resources to get you started.
I’ve gone on for a while now. Let’s bring this post to a close. If you’re starting out with PHP this year, I hope that the information and resources that I’ve presented help get you off to a great start. If you’ve been learning for a little while now, what resources would you add?
If you’re having trouble getting PHP’s Memcached extension working in a Docker container, this tutorial will show you how.
When you need to handle a 404 (not found) error in Mezzio, how do you do it? It’s trivial in Laravel, Symfony, CakePHP, and other, larger PHP frameworks. But, it’s not so clear in Mezzio. In this tutorial, I’ll show you three ways to do so.
Are you getting the most out of Composer? In this short tutorial, you’re going to learn about more of its functionality including removing and updating dependencies, and configuring autoload namespaces.
Composer is one of the best package managers available for any, modern, software development language. In this short tutorial, you’re going to learn the essentials of how to use it, so that if you’re just getting started with it, you can get up and running quickly.
Please consider buying me a coffee. It really helps me to keep producing new tutorials.
Join the discussion
comments powered by Disqus