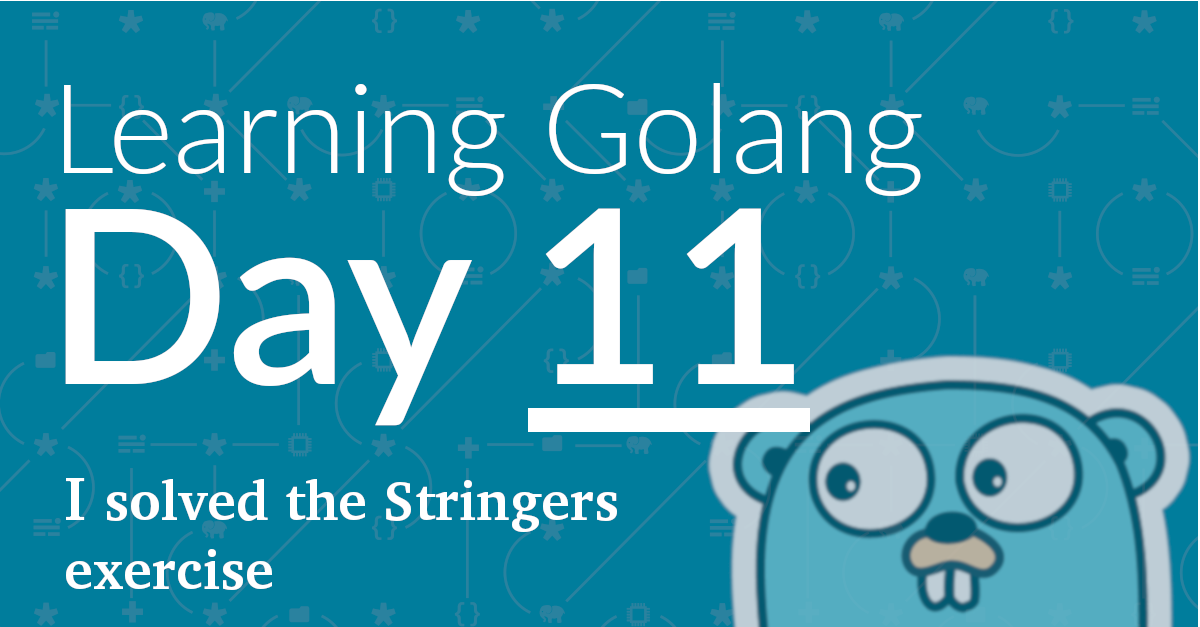
Learning Golang, Day 11 – I Solved the Stringers Exercise!
Here we are on day 11, where I solved the Stringers Exercise.
Here we are on day 10. Today I read about and played with Type Assertions, Type Switches, and Stringers in Go.
:idseparator: - :idprefix: :experimental: :source-highlighter: rouge :rouge-style: pastie :imagesdir: /images
I enjoyed the session, as it, on the whole, made sense. Type https://go.dev/tour/methods/15[assertions] and https://go.dev/tour/methods/16[switches] were pretty self-explanatory, and something that I look forward to using. The same goes for https://go.dev/tour/methods/17[Stringers], which remind me a lot of PHP’s https://www.php.net/manual/en/language.oop5.magic.php#object.tostring[magic __toString function].
Because of that comparison, they’re what I want to focus on for just a bit.
From what I understand, to implement a Stringer, all you have to do is to add a method to a Type in Go named String
that returns a string
.
In PHP, if a class implements the __toString
method, which returns a string, then the object can be used as a string.
Here’s an example to demonstrate what I’m talking about.
Here we are on day 11, where I solved the Stringers Exercise.
Today, on day 14, I created a custom method to remove code duplication creeping into the weather station codebase. Come read the fun story behind getting that done.
Here we are on day 13. Today, I continued learning Golang by working on the Golang version of my PHP/Python weather station, adding a function to render static pages. Let me share my learnings with you.
Here we are on day 12. I didn’t solve anything. I’m feeling that these exercises are becoming arbitrary and pointless.
Please consider buying me a coffee. It really helps me to keep producing new tutorials.
Join the discussion
comments powered by Disqus