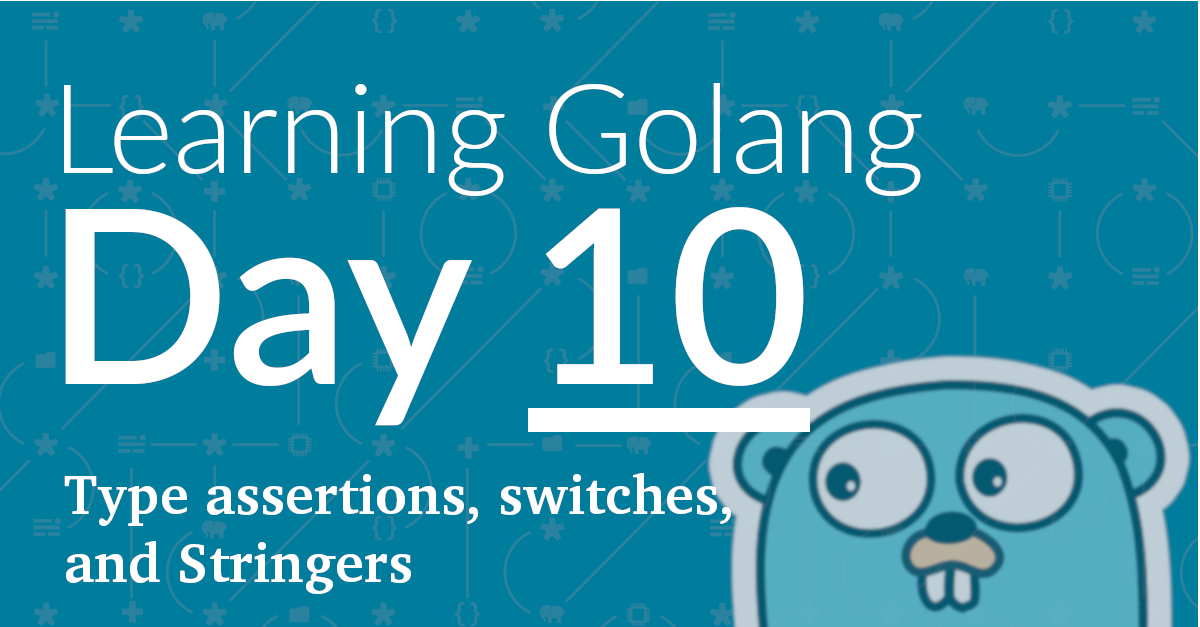
Learning Golang, Day 10 – Type Assertions, Type Switches, and Stringers.
Here we are on day 10. Today I read about and played with Type Assertions, Type Switches, and Stringers in Go.
Here we are on day 11, where I solved the Stringers Exercise.
:idseparator: - :idprefix: :experimental: :source-highlighter: rouge :rouge-style: pastie :imagesdir: /images
Well, while I did implement the solution today, it was yesterday when I felt that I was on the right track, encouraged by Peter Hellberg in the #newbies channel of https://gophers.slack.com[gophers.slack.com]. Thanks, Peter!
If you’ve not read https://go.dev/tour/methods/18[the problem definition], it’s:
Make the IPAddr type implement fmt.Stringer to print the address as a dotted quad. For instance, IPAddr{1, 2, 3, 4} should print as “1.2.3.4”.
Here’s my solution, which uses a combination of the append()
function, fmt.Sprintf()
, and strings.Join()
.
package main
import ( “fmt” “strings” )
type IPAddr [4]byte
func (ipa IPAddr) String() string { var output []string for _, v := range ipa { output = append(output, fmt.Sprintf("%d", v)) }
return strings.Join(output, ".")
}
I had a good think about several, possible ways that I could approach this, but kept thinking that Go had to have something analogous to https://www.php.net/manual/en/function.implode.php[PHP's implode() method].
If you’re not familiar with implode, it joins the elements of an array together with a given string.
So, the following example, would print out: James, Jodie, Matthew, Michael
.
Here we are on day 10. Today I read about and played with Type Assertions, Type Switches, and Stringers in Go.
Today, on day 14, I created a custom method to remove code duplication creeping into the weather station codebase. Come read the fun story behind getting that done.
Here we are on day 13. Today, I continued learning Golang by working on the Golang version of my PHP/Python weather station, adding a function to render static pages. Let me share my learnings with you.
Here we are on day 12. I didn’t solve anything. I’m feeling that these exercises are becoming arbitrary and pointless.
Please consider buying me a coffee. It really helps me to keep producing new tutorials.
Join the discussion
comments powered by Disqus