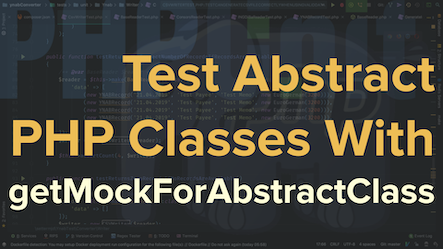
How to Test Abstract PHP Classes With PHPUnit
How do you test abstract classes in PHP? Find out how to test them in this post by using PHPUnit’s getMockForAbstractClass method.
If you love using data providers in PHPUnit, but find defining them verbose (and potentially buggy) then you’ll love the attribute-based approach in PHPUnit 10. Learn the essentials in this short post.
If this is your first time hearing about them, data providers allow you to invoke a test function multiple times, providing it with different data on each invocation. They’re an excellent – and simple – way of broadening your test coverage
Take a look at example below, and then let’s step through it together.
/**
* @dataProvider referenceIDDataProvider
*/
public function testCanValidateReferenceIDs(
string $referenceID,
bool $isValid
): void {
$validator = new MyHypotheticalValidatorClass();
$result = $validator->isValidReferenceID($referenceID);
($isValid)
? $this->assertTrue($result)
: $this->assertFalse($result);
}
public function referenceIDDataProvider(): array
{
return [
[
'MSAU2407240002',
false,
],
[
'MSAU2407240001',
true,
],
];
}
The test function (testCanValidateReferenceIDs
) takes two arguments:
$referenceID
, a string$isValid
, a boolIt passes $referenceID
to the subject under test ($validator->isValidReferenceID()
) and, based on the value of $isValid
, asserts whether the result of the function call ($result
) is true or false.
For the purposes of a simple example, assume that isValidReferenceID()
is a small function that checks whether $referenceID
matches two criteria:
As the @dataProvider
annotation is used in the test’s docblock, PHPUnit will then look for the function name specified (referenceIDDataProvider
) and iterate over the array that the function returns, passing the values of each inner array to an invocation of testCanValidateReferenceIDs()
.
So, the first iteration would receive 'MSAU2407240002'
and false
, and the second iteration would receive 'MSAU2407240001'
and true
.
That’s fine, but there’s a lot of boilerplate code to write.
And, the more arguments you pass to the test the more complicated the array returned from the dataProvider
function can become.
Consequently, while extremely versatile and flexible, it can become increasingly error prone – not to mention frustrating to maintain.
But, with the introduction of attributes in PHP 8, and their uptake in PHPUnit 10, this verbose approach becomes a thing of the past. Take a look at the revised test function definition below.
<?php
use PHPUnit\Framework\Attributes\TestWith;
#[TestWith(['MSAU2407240002', false])]
#[TestWith(['MSAU2407240001', true])]
public function testCanValidateReferenceIDs(
string $referenceID,
bool $isValid
): void {
$result = $this->handler
->isValidReferenceID($referenceID);
($isValid)
? $this->assertTrue($result)
: $this->assertFalse($result);
}
In this version you import the TestWith attribute. Then, you specify it once for each different data set that you want to invoke the test function with. There’s no separate function to write, and the structure is less complicated. This makes it easier to understand, clearer, and more maintainable.
Now, while the example above was a good introduction, what about a more complicated example, say where one or more parameters, – even both – is an array?
Is the TestWith
attribute still readable and a good idea?
Let’s have a look.
Let’s say that we have the following function that takes an array
<?php
use PHPUnit\Framework\Attributes\TestWith;
#[TestWith(
[
[
],
[
'content_html',
'from_address',
'from_name',
'subject',
'to_address',
'to_name',
],
]
)]
#[TestWith(
[
[
'from_address' => 'send.from@example.com',
'from_name' => 'Sender',
'to_address' => 'send.to@example.com',
'subject' => 'SendGrid Test Email',
],
[
'content_html',
'to_name',
],
]
)]
#[TestWith(
[
[
'from_address' => 'send.from@example.com',
'from_name' => 'Sender',
'subject' => 'SendGrid Test Email',
'to_address' => 'send.to@example.com',
'to_name' => 'Recipient',
],
[
'content_html',
],
]
)]
public function testReturnsJsonErrorResponseIfPostParametersAreMissing(
array $requestBody = [],
array $missingItems = []
): void
{
$request = $this->setServerRequest($requestBody);
$handler = new SendEmailHandler(
$this->mail,
$this->sendgrid
);
$response = $handler->handle($request);
$this->assertInstanceOf(
JsonResponse::class,
$response
);
$this->assertSame(
StatusCode::BAD_REQUEST,
$response->getStatusCode()
);
$this->assertSame(
[
"Error" => "Missing configuration items.",
"Missing configuration items." => $missingItems,
],
json_decode(
json: (string) $response->getBody(),
associative: true,
flags: JSON_OBJECT_AS_ARRAY
)
);
}
vendor/bin/phpunit \
--do-not-cache-result \
--filter testReturnsJsonErrorResponseIfPostParametersAreMissing#1
PHPUnit 12.0.5 by Sebastian Bergmann and contributors.
Runtime: PHP 8.4.4
Configuration: /Users/msetter/Workspace/PHP/Twilio/sendgrid-php-testing/phpunit.xml.dist
. 1 / 1 (100%)
Time: 00:00.027, Memory: 10.00 MB
OK (1 test, 4 assertions)
➜ sendgrid-php-testing git:(branch-step-through-changes) ✗ vendor/bin/phpunit --do-not-cache-result --filter testReturnsJsonErrorResponseIfPostParametersAreMissing
PHPUnit 12.0.5 by Sebastian Bergmann and contributors.
Runtime: PHP 8.4.4
Configuration: /Users/msetter/Workspace/PHP/Twilio/sendgrid-php-testing/phpunit.xml.dist
..... 5 / 5 (100%)
Time: 00:00.022, Memory: 10.00 MB
OK (5 tests, 20 assertions)
➜ sendgrid-php-testing git:(branch-step-through-changes) ✗ vendor/bin/phpunit --do-not-cache-result
PHPUnit 12.0.5 by Sebastian Bergmann and contributors.
Runtime: PHP 8.4.4
Configuration: /Users/msetter/Workspace/PHP/Twilio/sendgrid-php-testing/phpunit.xml.dist
.............. 14 / 14 (100%)
Time: 00:00.032, Memory: 10.00 MB
OK (14 tests, 71 assertions)
I appreciate that it may not, yet, be an option for you, as you may be stuck using a version of PHP prior to 8. Or, for reasons outside of your control, you may not be able to upgrade, or may be on a version of PHPUnit prior to 10.
If given the choice though, which approach would you use? Let me know in the comments.
How do you test abstract classes in PHP? Find out how to test them in this post by using PHPUnit’s getMockForAbstractClass method.
Substitutability or the Liskov Substitution Principle (LSP) is a concept that I’ve tried to adhere to for some years when writing code. It’s beneficial for many reasons, but particularly when testing, as it can indirectly force you to write code that is more testable. Recently, I’ve started appreciating how it works in Go, and will step through how in this short article.
Debugging requests can be a time-consuming process. However, there’s a tool that makes doing so a lot simpler. It’s called Postman. In this tutorial, I step you through its core features and show you how to use them.
PhpStorm offers so much functionality. From syntax highlighting to Docker integration, it’s an extremely comprehensive tool. However, have you ever thought of using it to run your unit tests? In this article, I step you through running tests, from an entire suite to an individual test.
Please consider buying me a coffee. It really helps me to keep producing new tutorials.
Join the discussion
comments powered by Disqus