If you’re getting started learning Java, whether because you want to or you have to, I want to help you out, as I’m learning Java again too. In this post, I set out what I think you need to know to get started with it, or what your first few steps will likely entail.
Recently, I’ve been working with a friend of mine, who’s a mature-age University student.
If the term “mature-age” isn’t familiar, it’s the term we use in Australia for people who don’t go to university directly out of school, but rather after a handful of years after leaving school.
He’s working through a Bachelor of Information Technology, something I can intimately relate to, as I completed the same degree (from the same institution).
As a result, we’ve had some riveting discussions about what the experience has been like so far, the curriculum, how helpful the faculty staff are — & so much more!
One particular point of discussion has been that he’s learning about software development through the lens of Java.
That’s the language, rightly or wrongly, that the Computer Science faculty at his university use to teach software development.
As a side note, Java is the language that the computer science faculty used to teach software development when I studied for my undergraduate degree.
Frustratingly, he’s been hitting some stumbling blocks recently while learning Java.
Combine that with the fact that I’ve not used Java a whole lot over the intervening years since I left university, but had always “meant to”, I thought I’d take the opportunity to dive on back in and start relearning Java, in a bid to help him out.
I’ve learned so much over the last few weeks.
I’ve been pleasantly surprised that so many of the stories that I’ve heard associated with Java, such as it being bloated (or at the least, extremely verbose) either no longer hold or aren’t as relevant as they once were.
In this post, I’ll outline what I see as the key things you need when you’re starting out learning Java.
Whether you’re a complete beginner to Java, or someone returning to it, after a long hiatus, there’ll be something for you.
If you’re a Java geek, I’d love to hear if you can relate to these experiences, or if you completely disagree with them, in the comments below.
What Java Has Going For It
Java Has Excellent IDE Support
As you’d expect of a language regularly touted as an “enterprise” language, it has excellent IDE support; actually, some of the best that I’ve seen.
I’ve been using IntelliJ IDEA Community Edition (2019.3).
But there are other options available, including Eclipse and Netbeans.
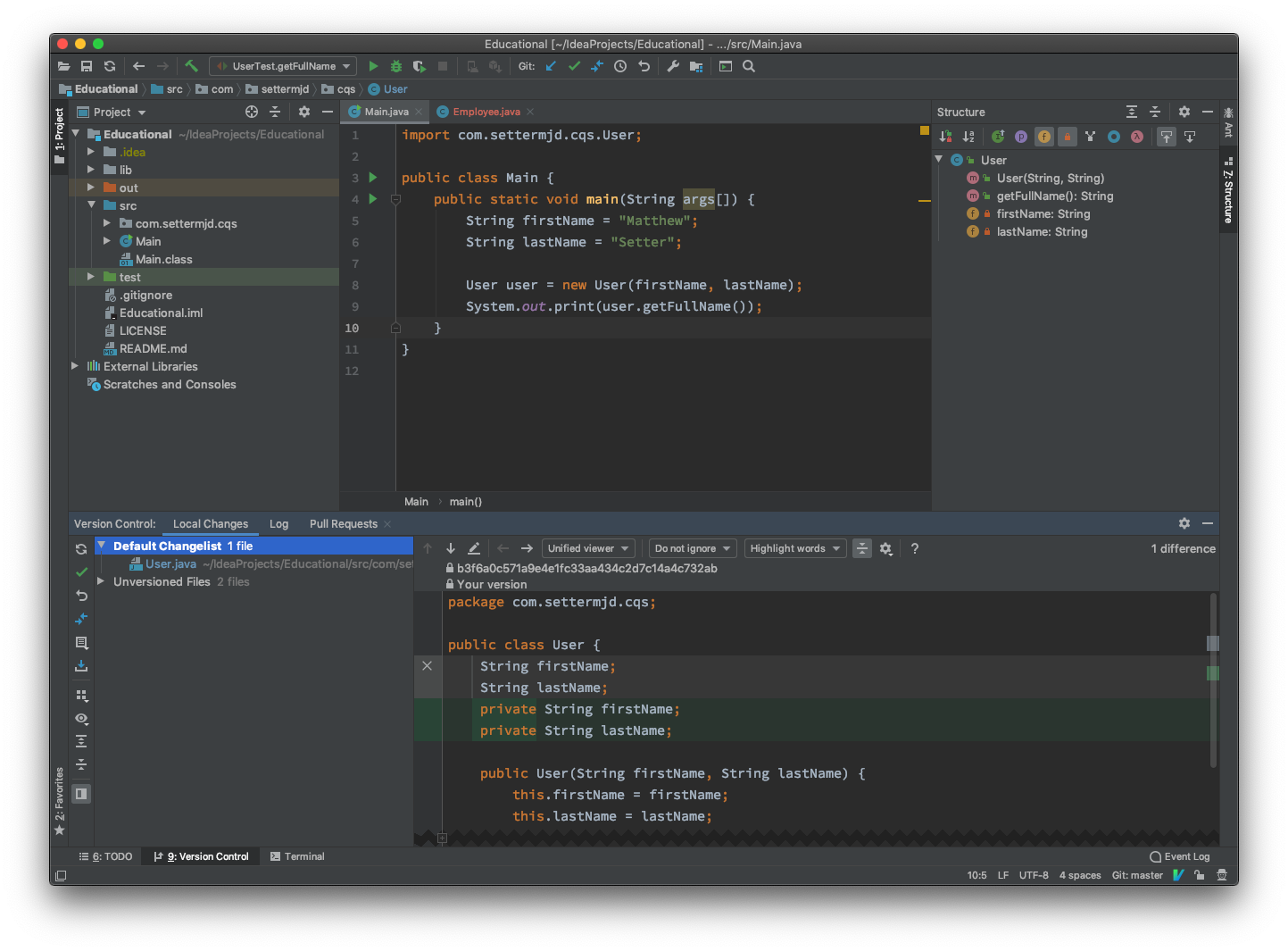
Depending on which one you choose, they have a wealth of functionality that makes the developer experience so meaningful.
This functionality includes:
- Code editing
- Code insight
- Debugging
- Diff and merge
- GUI development
- Polyglot language support
- Profiling; and
- Refactoring
These editors also provide integration with related tools, such as Docker, Git, documentation tools (such as Markdown and AsciiDoc, and external services.
A lot of this functionality is provided out of the proverbial box.
But they also have a plugin infrastructure that supports extending them with a range of additional functionality.
This includes additional, Java-related languages, such as Scala, Clojure, Groovy, and Kotlin.
It can be integrated with build tools, such as Ant, Maven, and Gradle.
It can be integrated with testing frameworks, such as JUnit.
It can compile your code, avoiding the need to drop to the command-line.
No matter what your needs are, your IDE likely, has you covered.
Excellent Text Editor Support
Now not everyone likes using IDEs.
Some feel that they’re bloated.
Some feel that they’re unnecessary.
Some feel that they hide things from you.
Some feel that they make developers lazy.
Do they?
Really?
I don’t believe so.
Other people just prefer text editors.
There are a lot of good reasons for that preference, which I’ll go into another time.
If you’re that kind of person, then there are packages, extensions, and plugins for many text editors, such as VIM, Emacs, SublimeText, Atom, and Visual Studio Code that will give you a productive Java development environment.
Excellent Automation/Build Support
Then there are the excellent automation packages, that help ensure you can do almost anything in a repeatable way.
Whether that’s running your tests, building your software, validating your code, or anything in between, you’re covered.
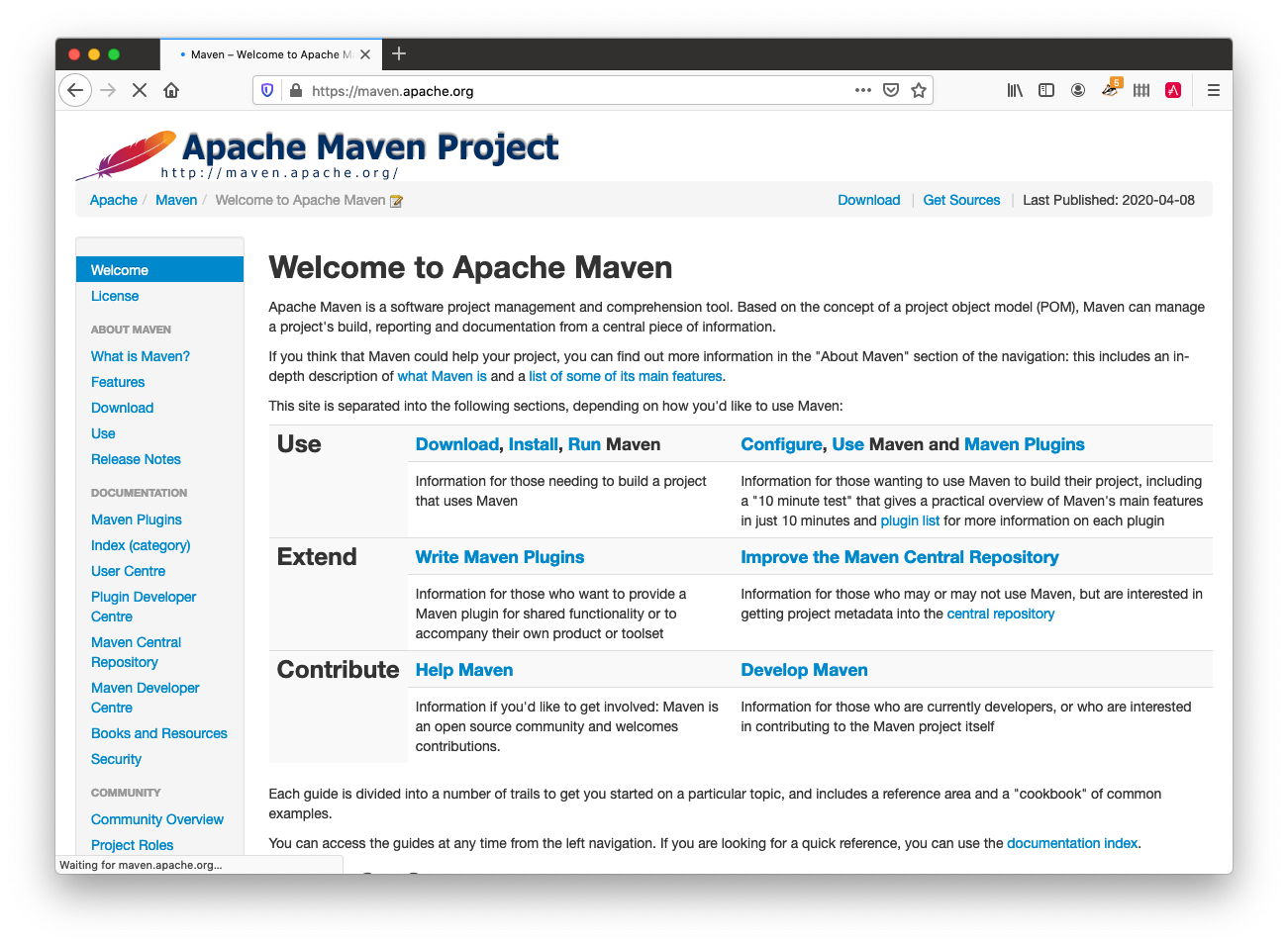
Three of the best are Ant, Maven (my personal favourite), and Gradle.
They can be used for any software language; such as PHP, Python, C/C++, and C#.
However, they’re really focused on the JVM—especially Java.
Excellent Documentation Available
Java has been used extensively for many years, in countless industries, and across many countries around the world.
As a result, there’s a wealth of information available to learn just about aspect of it.
There are courses, books, blog articles, podcasts, and more.
Libraries and Frameworks
The final benefit I’m going to mention, is that Java has libraries and frameworks for pretty much any problem you need to solve.
Whether you’re building a web-based application or API, a small or large GUI application, an embedded application, a microservice, or something else entirely, Java has you covered.
Here is a small selection to get you started.
But, What’s It Like After a Hiatus or For a Junior Developer?
The points that I’ve just covered are but the tip of the proverbial iceberg.
And, from the way that I’m writing this post up until now, you’d think that I was a Java fanboy.
I’m not, but you could be forgiven for thinking so.
When I started getting back into Java, I was very frustrated with the experience.
I started to wonder why anyone would bother using the language when you had to, seemingly, use so much tooling just to write a stereotypical Hello World application with tests.
For example:
- Why did it seem necessary to create an intricate directory structure?
- Why did it seem necessary to use a build tool?
- Why couldn’t I just pop open VIM, write one, small, file, and then run a reasonably short bash one-liner to get the app compiled and run?
Well, you can.
And when I had enough patience to set my assumptions and biases aside, I remembered that it is possible.
If you have a look at the code below, you can see a “Hello World” example.
public class Main {
public static void main(String args[]) {
System.out.println("Hello World");
}
}
To run it, after installing a recent copy of OpenJDK, I only needed to run two commands:
javac Main.java && java Main
So, that put paid to some of my initial thoughts.
To be fair, I could barely create and execute the equivalent in PHP, bash, Python, or Ruby any faster than it took me to write the Java example above.
Yes.
It seems no harder to start writing Java now than it did when I first learned it — back in 1997.
Yes, I did say 1997.
However, that previous example was just a trivial code example.
It’s not going to:
- Win anyone any prizes
- Control the Mars Rover; or
- Perform climate modelling calculations
It’s just a small example to prove a point.
If I wanted to write a more feature-rich application, I’d need to apply a number of concepts and use a bit of tooling, such as the aforementioned Maven and JUnit.
After all, if you think that writing code doesn’t involve tests, then you’re no better than a script kiddie.
What Does it Take to Get Started Learning Java?
There are so many things that you could do.
But, what is the absolute minimum that you need to do?
After putting quite some thought into this, here’s what I believe you need to know (or be willing to learn).
1. The Fundamentals of Object-Oriented Programming (OOP)
If someone new to Java has sufficient experience in writing code, if they’re familiar enough — or prepared to become familiar enough — with Object-Oriented Programming, then they can learn Java.
If not, get to know classes, objects, encapsulation, delegation, and inheritance.
That said, Java’s default access modifier did trip me up for a little while, until I’d rammed the concept into my head a bit, and experimented with it.
It seems like an odd implementation detail at this point.
I’m guessing, as with annotations, with further reading I’ll come to understand it fully.
One final point, we can leave the often rather lengthy discussions about what OOP truly is — and what it isn’t — for another time.
Here’s a good start from Robert C. “Uncle Bob” Martin.
2. What Packages Are
You need to know what packages are and why you should use them.
If you’re not familiar with them, here’s a short intro from GeeksforGeeks;
Packages:
- Prevent naming conflicts
- Make searching/locating and usage of classes, interfaces, enumerations and annotations easier
- Provide controlled access: protected and default have package level access control
- Can be considered as data encapsulation (or data-hiding)
3. How to Organise Your Code
You need to know how to organise the source, and related, files that your project will require with them.
Proper file organisation isn’t specific to Java.
Any language, any good project actually, requires a high degree of proper organisation.
4. How to Compile and Build an App by Hand (Without the Support of an IDE)
I know that I’ve talked a bit about build tools, but you need to know what they do for you, such as building and executing an app by hand.
To do that, you need to appreciate what Path and Classpath are.
You need to know how to set them when compiling and running your apps.
There are many build tools available for Java, two of the most popular being Maven and Gradle.
However, regardless of the tool that you choose, you need to know the following four things:
- What a build tool is
- The benefits of using a build tool
- The basics of how to configure them
- How they structure their directories and files. Here’s an introduction to Maven’s standard directory layout; and
- The key commands to use them
The Basics of Testing
Specifically, you need to know:
- What testing is
- What a testing framework is
- The benefits of using testing and a testing framework when building quality software; and
- The basics of how to get one working with your code
There are many different types of testing used in modern software development, including unit, functional, integration, and smoke testing.
Then, at a higher level, there are different testing methodologies, such as Test-Driven Development (TDD), and Behavior-Driven Development (BDD).
If you’re coming to testing for the first time, or are quite new to it, you could easily feel quite confused as to where to start.
So, here’s my advice: start with unit tests.
To do that, JUnit has you covered.
Once you’re familiar with JUnit, then, if you need to, branch out from there, to other frameworks and tools, such as Selenium for Java UI testing, Mockito, Spock, and Cucumber.
Know What Annotations Are
If I were a Java-hater, I’d likely start to say things like, why are annotations necessary in a modern programming language?
If I were an annotation-hater, I’d definitely say it.
For the record, I’ve never had any hatred for annotations.
Where they’ve helped, I’m all for them — like most things.
Without having read more about them, or the motivations for implementing them, I can only infer that they help the JVM as well as the developer express intent that much more clearly, in a way that has not, as yet, been able to be implemented in a better way.
The following snippet from GeeksforGeeks does an excellent job of clarifying them:
Annotations help to associate metadata (information) to the program elements, i.e. instance variables, constructors, methods, classes, etc.
Annotations are not pure comments as they can change the way a program is treated by the compiler. See below code for example.
Where To From Here?
I would feel deeply remiss if I didn’t provide a host of additional resources for learning java, so that, as the saying goes, you can help those who help themselves.
So, here are a host of resources that I’m using, or plan to use, in my Java journey.
I hope that they help you as well.
IRC
Forums
Podcasts
Here is a selection of 25 podcasts all about software development.
Documentation
You’re going to need to refer to the Java documentation, so here is the latest version (at the time of writing).
Developers to Follow
If you’re looking for some great Java-focused/experienced developers to follow, here is a small selection of the ones that I am familiar with.
Books
If you’re someone who prefers a good read and to learn by reading, then here is a small selection of books to get you going.
The first two are focused purely on Java.
The second two are for software development more generally.
I highly recommend all four.
In Summary
I’ve gone on for a while now.
Let’s bring this story to a point.
Java Still Feels, well, “Different”.
If you’re a polyglot developer, a developer who’s been writing code for some years, then the points that I’ve listed above may not sound in any way unique.
Arguably, if I were starting to learn PHP, Go, Python, Ruby, C/C++/C#, or any other modern software development language, I’d likely write, more or less, the same list.
But there’s something about Java, that seems to necessitate a longer, more detailed list of requirements.
Perhaps it’s just because I’ve gotten a flawed perception stuck in my head.
I’m not sure.
But, to me at least, Java just seems to need more tooling to help you automate away a lot of the requirements that it places on you to create high-quality software — more so than other languages.
If I’m honest, and I am, the days of writing, building, and deploying high-quality code by hand are long gone.
Modern software has so many demands placed on it, has so many high expectations of it that you can’t do it all on your own, even if you are a legend in your own lunch box.
That’s My Take On Learning Java in 2020
Java is a language that has a whole lot of unnecessary baggage wrapped around it, perhaps mainly because of Oracle.
But despite that, it’s a language that serves a lot of valid needs.
As a result, it’s worth learning if you want to learn it.
It will take time to get going with it, but then, anything worthwhile takes time.
These have been my thoughts on what you’re going to need to do, as a minimum, to start learning Java.
I’d love to know what you think if you’re a more seasoned Java developer.
P.S., as a continuation of this article, I’ve set myself the goal of getting a Java role within the next 3 months, so I’m actively following the advice that I’ve laid out in this article.
If you want to follow my journey (perhaps we could compare notes) then I’ll be tweeting under the hashtag #mattsjavajourney
and writing posts tagged with Java.
Join in, if you’re getting in to Java for the first time, or back in to it, like I am.
If you’re just starting learning Java or starting again, I’m currently working on a free Java Beginners course (the title is currently in flux).
If you’re interested in taking the course, sign up to be informed about course progress and to know when it is released.
I’m aiming for the end of July this year, at this stage.
Join the discussion
comments powered by Disqus