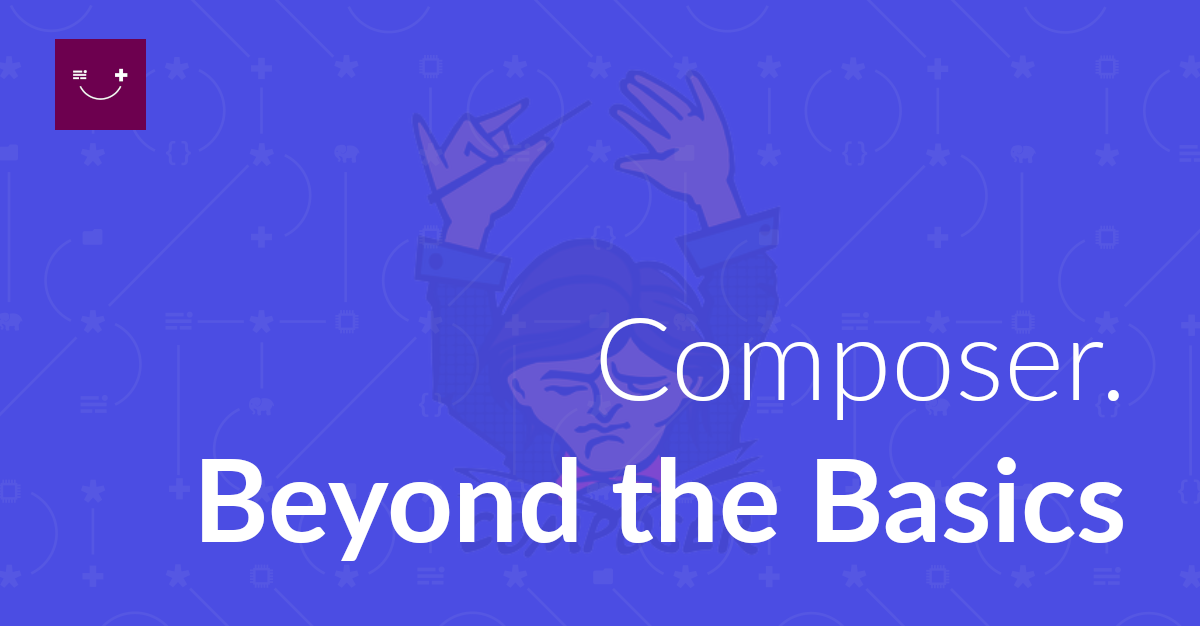
Composer. Beyond the Basics
Are you getting the most out of Composer? In this short tutorial, you’re going to learn about more of its functionality including removing and updating dependencies, and configuring autoload namespaces.
Is PHP worth learning in 2025, with all of the attention that other languages, such as Go, get? In this post, I’ll show you a series of compelling reasons why PHP is still worth learning - even if it’s not adopted as much nor as quickly as it once was.
PHP continues to grow and mature with each new release - especially since the 7.x series. So much so that, for me, it’s now the language that I wish it was when I started using it in the early 3.x days, back around 2000.
It might seem hard to believe, but in those days OOP (object-oriented programming) constructs were syntactic sugar. Now, they’re baked into the language. Not only that, but there are Typed Class Constants, Attributes, Union Types, Intersection Types, Property Hooks, and so much more.
Yet, despite this continued growth and development, where other languages, such as Perl, have faltered over the years, people have continued to claim (and hope) that PHP is dying – and that that time can not come soon enough.
An excellent example is The PHP Singularity by Jeff Atwood founder of StackOverflow, written back in 2012.
Consider how the post concludes:
From my perspective, the point of all these “PHP is broken” rants is not just to complain, but to help educate and potentially warn off new coders starting new codebases. Some fine, even historic work has been done in PHP despite the madness, unquestionably. But now we need to work together to fix what is broken. The best way to fix the PHP problem at this point is to make the alternatives so outstanding that the choice of the better hammer becomes obvious.
That’s the PHP Singularity I’m hoping for. I’m trying like hell to do my part to make it happen. How about you?
Then there’s the classic “PHP: a fractal of bad design”. Quoting LWN.net, as the original post seems to be deleted:
It’s a bit of a rant, but a blogger known as “Eevee” has put together a detailed criticism of PHP as a language. It covers the flaws Eevee sees in the predictability, consistency, reliability, debug-ability, security, and many other attributes of the web application language. “PHP is the lone exception. Virtually every feature in PHP is broken somehow. The language, the framework, the ecosystem, are all just bad. And I can’t even point out any single damning thing, because the damage is so systemic. Every time I try to compile a list of PHP gripes, I get stuck in this depth-first search discovering more and more appalling trivia. (Hence, fractal.)”
I’ve seen that post quoted more than any other PHP hack piece over the years.
So, given the evolution in the language and the continued hate pile on, is it worth learning PHP in 2025 – especially from a career perspective? Well, consider PHP has going for it.
Unlike languages such as Java, PHP takes trivially little time to get up and running, and requires virtually no tooling to do so either! You only need three things:
Now, let’s look at a simple example. Let’s say that you want to redirect requests from one domain to another. Here’s one way to do it:
<?php
declare(strict_types=1);
function redirectToNewDomain(string $oldDomain, string $newDomain): void
{
$requestUrl = sprintf(
"%s://%s%s",
$_SERVER["HTTPS"] === true ? "https" : "http",
$_SERVER['HTTP_HOST'],
$_SERVER["REQUEST_URI"]
);
if (parse_url(filter_var($requestUrl, FILTER_SANITIZE_URL), PHP_URL_HOST) === $oldDomain) {
header("Location: " . $newDomain, true, 301);
exit;
}
}
$baseDomain = "deploywithdockercompose";
redirectToNewDomain(
sprintf("%s.com", $baseDomain),
sprintf("https://%s.webdevwithmatt.com/", $baseDomain),
);
With nothing more than the functionality available in standard PHP, including its superglobals and internal functions, you’re able to do so. Now, in your terminal, you can run the code with the command below.
php -S 0.0.0.0:8080 -t ./
Assuming that you stored the code in a file named index.php in the current directory, this command would start PHP’s built-in web server listening for requests on port 8080, and passing them to index.php.
Sure, the example above is a little contrived, but it’s something I’ve been working on, in a more sophisticated way, recently). Regardless, it still serves to show just how easy it is to get up and running with PHP.
Want a more sophisticated example? Then check out the tutorial I wrote (for the Twilio blog) which shows how to create a Markdown blog in PHP With the Slim Framework; plus the Standard PHP Library (SPL), Twig, and several other packages.
While there are other IDEs, perhaps the best example is PhpStorm. With its rich functionality and very polished user interface, it’s trivial for new PHP developers to get up and running quickly.
It has smart code completion, refactoring support, syntax error detection, type inference, excellent navigation throughout a codebase, and support for major PHP frameworks and tools including Laravel, Symfony, and WordPress.
But, other IDEs such as Eclipse and NetBeans also have a wealth of functionality that makes the developer experience so meaningful. This functionality includes:
These IDEs also integrate with tooling in the broader PHP ecosystem such as Docker, Git, and documentation tools.
A lot of this functionality is provided out of the proverbial box. But the IDEs also have a plugin infrastructure that supports extending them with a range of additional functionality.
Not everyone likes using IDEs; let’s be honest. Some feel that they’re bloated, unnecessary, that they hide things from you, and that they make developers lazy. Do they? Really?
But, if you prefer text editors you’re not short on choice, with such well-rounded options as:
These will all give you a super-productive PHP development environment very quickly. Sure, you will likely have to do more (at least initially) but from using Visual Studio Code for PHP and Go development over the last 12 months, it can be worth it.
If you’re a Laravel developer, you’re really spoiled for choice. An official Visual Studio Code Laravel extension is in the works. But, until then, you can use the Laravel Extension Pack or six great plugins, as reported by Laravel News.
While it’s trivially easy to get up and running with PHP, you have to build and deploy the application at some point. PHP is not short of options, only constrained by your budget, needs, and time to learn them. Some excellent choices are Phing, GitHub Actions, GitLab CI/CD, and Travis CI.
And, if you’re a Laravel developer, you can use Forge and Envoyer. Okay, they are able to deploy any PHP application, but I most commonly think of them in tandem with Laravel, as that’s where I most often hear them talked about.
Anyway, of these, I’m most familiar with GitHub Actions and GitLab CI/CD. With them you can build simple to sophisticated CI/CD pipelines, automating your deployments.
Unlike languages such as Go, which has formatting, linting, and testing as part of the language’s core tooling, PHP doesn’t. But, PHP has an array of packages available that provide that functionality, for example:
Given that these are third-party packages, it takes a little more time to get QA tooling set up for your PHP projects and packages, but not too long.
Okay, so I don’t have the most amount of experience with the official documentation of every software development language, but I’ve used it for numerous languages, such as Go, Ruby, and Python. Personally, I feel that the official PHP documentation is easily as good as any other.
Available in 11 languages, it covers anything that you may need to know about the language. You can find information on functions, types, variables, operators, exceptions, sessions, cookies, handling file uploads, and so much more. To me, the site has an excellent structure, is consistent throughout, and is easy to search. Well, the search could be just that much better, but it’s already pretty good.
PHP has libraries for pretty much any problem you need to solve, and a wealth of frameworks for building most any type of application. Whether you’re building a web-based application or API, a micro service – or something else entirely – PHP likely has you covered.
Here are some examples off the top of my head:
I could go on, but I think you get the point.
Now, let’s consider frameworks. Whether you want to build a small, one-page application, or a sophisticated service serving millions of requests a day, such as Fathom Analytics, PHP has a framework to suit your needs.
Sure, the majority of the market and mind-share, these days, goes to Laravel, which needs no introduction. But there are a wealth of others too, including Slim, Mezzio, CakePHP, Symfony, and Drupal.
Speaking from personal experience, I’ve used Slim, Mezzio, Drupal, and Symfony to build a range of websites and web-based applications with a minimum of fuss and effort. To differing degrees, they all have a wealth of in-built functionality including (but not limited to):
What’s more, they can be readily expanded upon as and when required.
I say that unashamedly. Composer is, hands down, one of the best package managers available in modern software development languages. What’s more, it is one of the key things that both kept PHP alive and ensured it became the language it is today!
Before the advent of Composer (first released on March 1, 2012), you either had to litter your code with require()
or require_once()
statements, or hand-craft your own autoloader, after autoloading was added to the language.
I found this pretty frustrating and tedious, not to mention an error-prone process at times.
After Composer came along, whether you used third party packages or not, you no longer needed to directly manage autoloading.
You could search for third party packages with composer search
and add them to your project with composer require
.
Then, you just needed to include Composer’s auto-generated autoloader (vendor/autoload.php) in your project and you had access to all of the classes, interfaces, functions, and traits that you needed, beautifully organised through Namespaces.
It was a proverbial godsend in how much time and effort it saved. Sure, no package manager is perfect. Even today there can be conflicts that prevent a package or a package version from being installed. But Composer is pretty good at telling you what the conflict is and helping you resolve it.
I could go on at length about just how good Composer is, but I don’t want to come across like a complete fanboy. What’s more, Composer has excellent documentation, and it’s functionality is a topic for another tutorial (series).
I could go on at length, talking about all of the reasons why PHP is worth using, why it’s a modern programming language, and how it’s ecosystem makes it a compelling choice. However, that would take more time to write than I have, and perhaps be somewhat pedantic.
So, instead, I’ll briefly list here a number of other compelling reasons:
PHP is a language that has a whole lot of unwarranted (and unnecessary) baggage attached to it; baggage which should have been left behind and forgotten about as it’s grown and matured over the years. Sadly, some can’t seem to find it within themselves to do that.
But, for the rest of us – and hopefully you – it’s a language that is extremely capable, with a rich ecosystem and community, one that can be put to use to serve an incredibly diverse range of needs. As a result, it’s worth learning if you want to learn it, or believe it will satisfy your use case. It won’t take you much time to get going with it either. Mastery, however, is different. But, anything worthwhile takes time.
These have been my thoughts on whether it’s worth learning PHP in 2025. I’d love to know what you think.
Are you getting the most out of Composer? In this short tutorial, you’re going to learn about more of its functionality including removing and updating dependencies, and configuring autoload namespaces.
Composer is one of the best package managers available for any, modern, software development language. In this short tutorial, you’re going to learn the essentials of how to use it, so that if you’re just getting started with it, you can get up and running quickly.
I recently refactored parts of a web application for an upcoming Twilio tutorial into three open source packages. Here’s what motivated the decision to do so, and why I think you should create them too.
Do you need to set environment variables for Composer scripts to work? Here’s how.
Please consider buying me a coffee. It really helps me to keep producing new tutorials.
Join the discussion
comments powered by Disqus